Guassian Elimination in C++
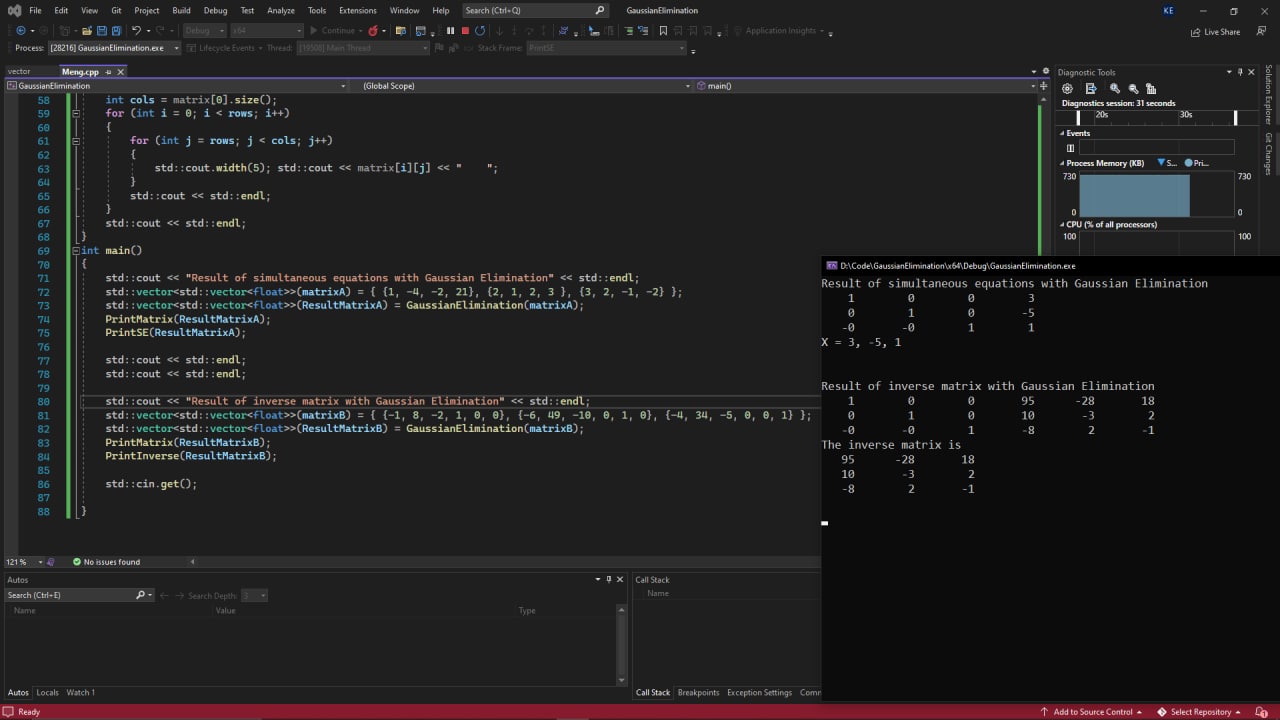
Calculate guassian elimination of any matrix size to solve simultineous equations and so on
04 Oct 2022
mathc++
Introduction
This program can calculate Guassian Elimination of any matrix size. It can solve simultaneous equations, inverse matrices and much more.
Code
/*28/09/2022 By Eong Koungmeng*/
#include <iostream>
#include <vector>
std::vector<std::vector<float>> GaussianElimination(std::vector<std::vector<float>> matrix)
{
int rows = matrix.size();
int cols = matrix[0].size();
for (int i = 0; i < rows; i++)
{
float coefficient1 = matrix[i][i];
for (int j = 0; j < cols; j++)
{
matrix[i][j] /= coefficient1;
}
for (int j = 0; j < rows; j++)
{
if (j == i) continue;
float coefficient2 = matrix[j][i];
for (int k = 0; k < cols; k++)
{
matrix[j][k] -= coefficient2 * matrix[i][k];
}
}
}
return matrix;
}
void PrintMatrix(std::vector<std::vector<float>> matrix)
{
for (int i = 0; i < matrix.size(); i++)
{
for (int j = 0; j < matrix[0].size(); j++)
{
std::cout.width(5); std::cout << matrix[i][j] << " ";
}
std::cout << std::endl;
}
}
void PrintSE(std::vector<std::vector<float>> matrix)
{
std::cout << "X = ";
for (int i = 0; i < matrix.size()-1; i++)
{
std::cout << matrix[i][matrix[0].size()-1] << ", ";
}
std::cout << matrix[matrix.size() - 1][matrix[0].size() - 1];
std::cout << std::endl;
}
void PrintInverse(std::vector<std::vector<float>> matrix)
{
std::cout << "The inverse matrix is\n";
int rows = matrix.size();
int cols = matrix[0].size();
for (int i = 0; i < rows; i++)
{
for (int j = rows; j < cols; j++)
{
std::cout.width(5); std::cout << matrix[i][j] << " ";
}
std::cout << std::endl;
}
std::cout << std::endl;
}
int main()
{
std::cout << "Result of simultaneous equations with Gaussian Elimination" << std::endl;
std::vector<std::vector<float>>(matrixA) = { {1, -4, -2, 21}, {2, 1, 2, 3 }, {3, 2, -1, -2} };
std::vector<std::vector<float>>(ResultMatrixA) = GaussianElimination(matrixA);
PrintMatrix(ResultMatrixA);
PrintSE(ResultMatrixA);
std::cout << std::endl;
std::cout << std::endl;
std::cout << "Result of inverse matrix with Gaussian Elimination" << std::endl;
std::vector<std::vector<float>>(matrixB) = { {-1, 8, -2, 1, 0, 0}, {-6, 49, -10, 0, 1, 0}, {-4, 34, -5, 0, 0, 1} };
std::vector<std::vector<float>>(ResultMatrixB) = GaussianElimination(matrixB);
PrintMatrix(ResultMatrixB);
PrintInverse(ResultMatrixB);
std::cin.get();
}
Result
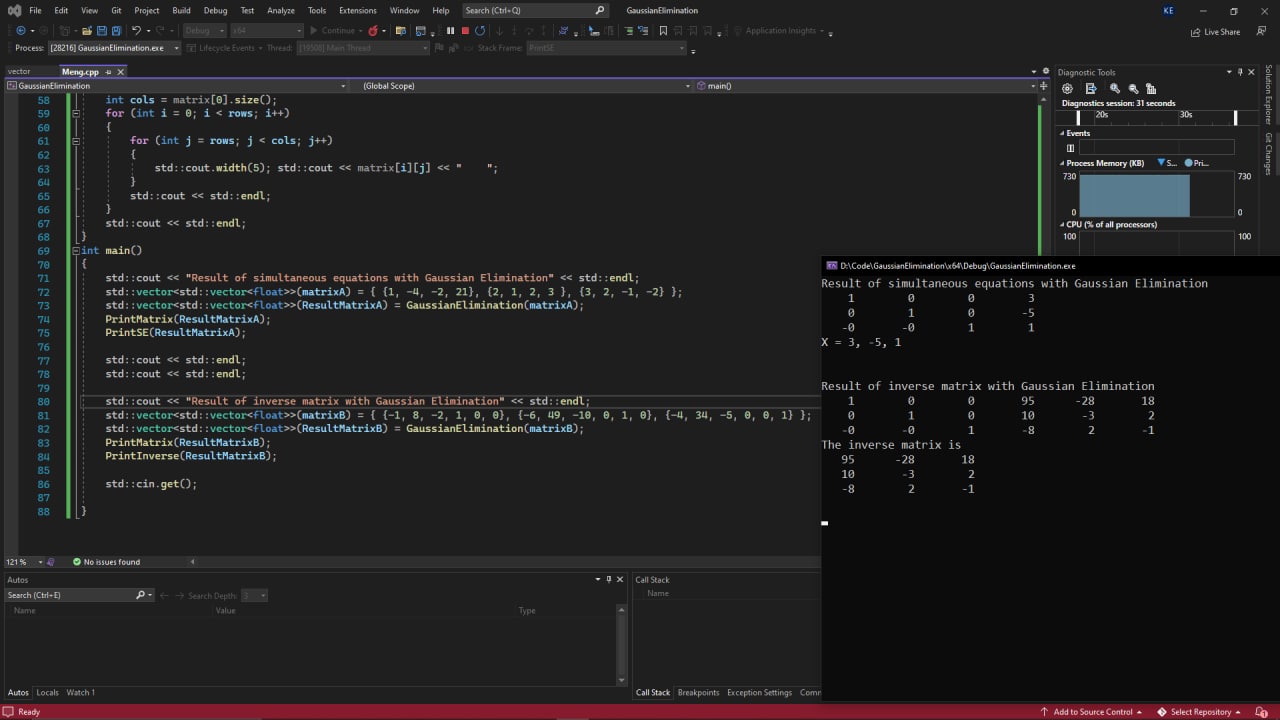
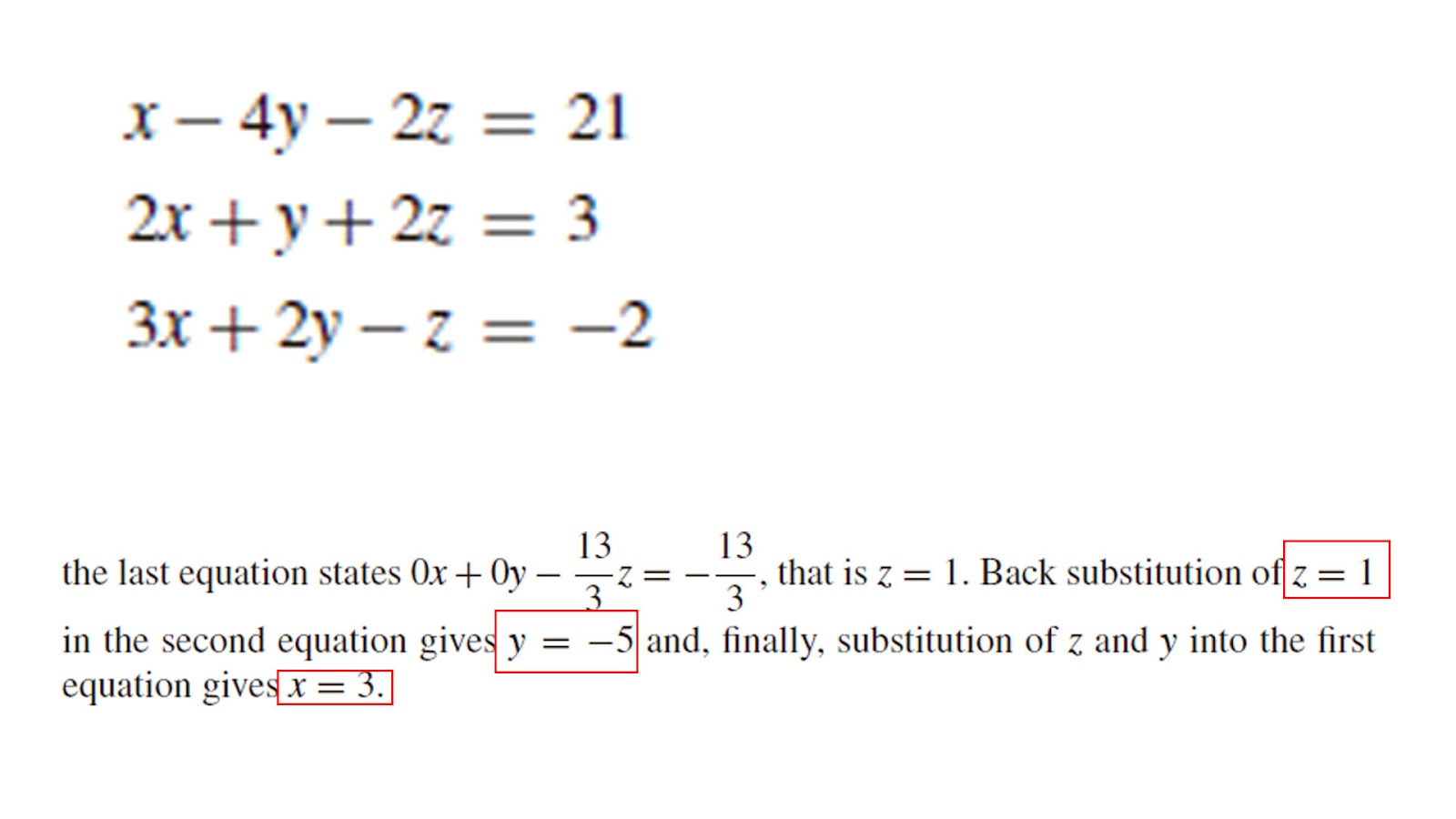
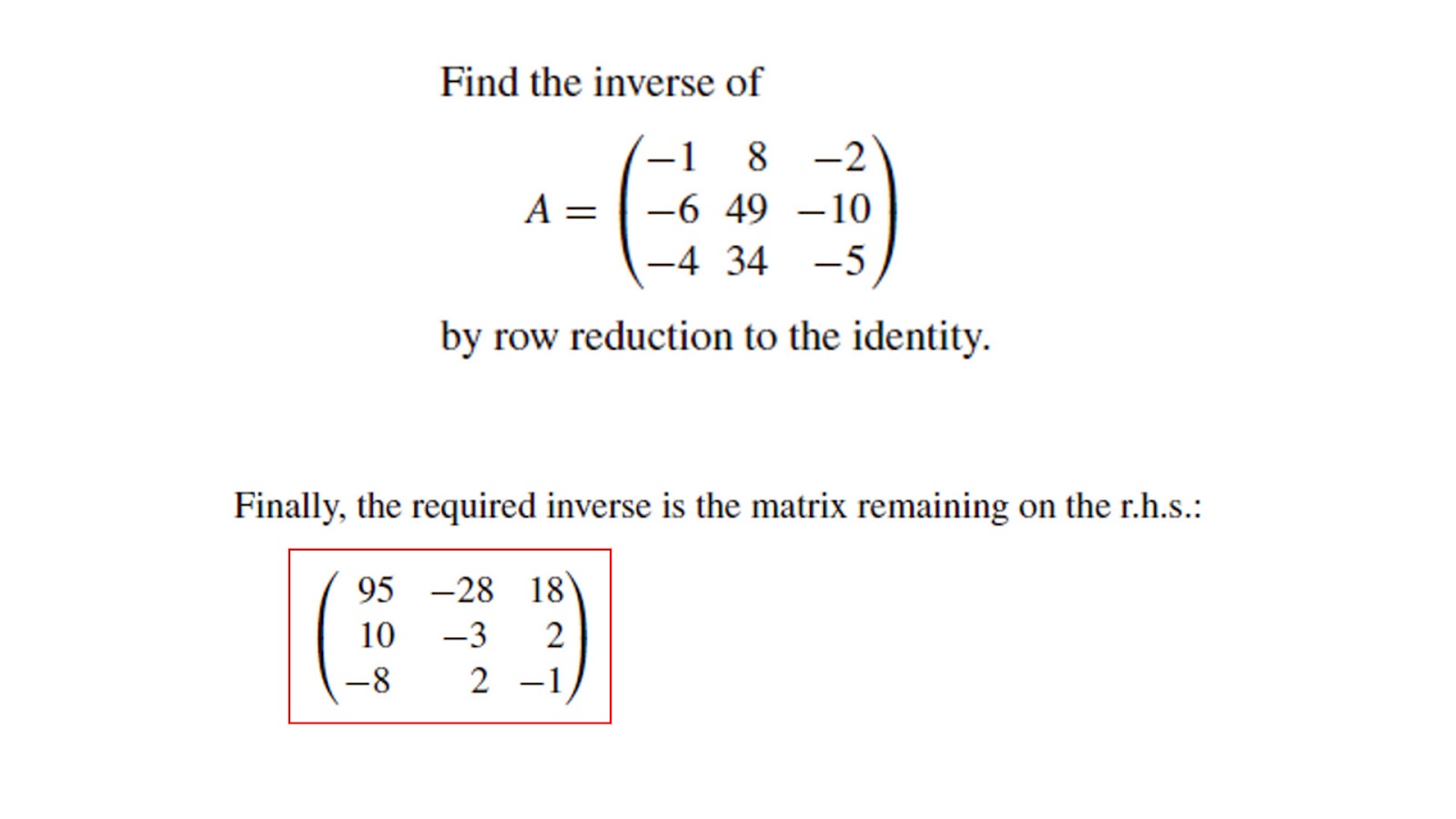